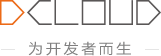
简体中文
每个组件都有属性和事件。有些属性和事件,是所有组件都支持的。
名称 | 类型 | 兼容性 | 描述 |
---|---|---|---|
id | string(string.IDString) | 组件的唯一标识。需避免同页面中不同组件设置重复id;需避免使用uni-、uni.等前缀 | |
style | string | UTSJSONObject | Array<string | UTSJSONObject> | 组件的内联样式,可以动态设置的内联样式 | |
class | string(string.ClassString) | UTSJSONObject | Array<string(string.ClassString) | UTSJSONObject> | 组件的样式类,在对应的 css 中定义的样式类 | |
ref | string | Function | vue中组件的唯一标识,用来给子组件注册引用信息,详见 | |
data-* | any | 自定义属性,组件上触发的事件时,会发送给事件处理函数 | |
android-* | any | App-Android平台专有属性,详见App-Android平台专有属性章节 |
android-开头的属性名称为App-Android平台专有属性
不支持动态修改此属性
更多信息可参考Android官方文档硬件加速。
App-Android平台设置组件视图渲染模型,字符串类型,可取值:
Tips
hardware
hardware
android-layer-type
为hardware
或software
时,overflow: visible
不生效。注意
4.61+
style
支持 UTSJSONObject
类型。Template
Script
<template>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view>
<page-head :title="title"></page-head>
<view class="uni-padding-wrap">
<view :id="generalId" :class="generalClass" :name="generalName" :title="generalTitle" :data-test="generalData"
:style="generalStyle" ref="general-target">
<text>id: {{ generalId }}</text>
<text>class: {{ generalClass }}</text>
<text>name: {{ generalName }}</text>
<text>title: {{ generalTitle }}</text>
<text>data-test: {{ generalData }}</text>
<text>style: {{ generalStyle }}</text>
</view>
<view class="btn btn-style uni-common-mt" @click="validateGeneralAttributes">
<text class="btn-inner">{{ validateGeneralAttrText }}</text>
</view>
<view class="btn btn-ref uni-common-mt" @click="changeHeight">
<text class="btn-inner">{{ changeHeightByRefText }}</text>
</view>
<view class="view-class" :hover-class="hoverClass" ref="view-target">
<text class="text">按下 50 ms 后背景变红</text>
<text class="text">抬起 400 ms 后背景恢复</text>
</view>
<view class="view-class" :hover-class="hoverClass" :hover-start-time="1000" :hover-stay-time="1000"
ref="view-target">
<text class="text">按下 1000 ms 后背景变红</text>
<text class="text">抬起 1000 ms 后背景恢复</text>
</view>
</view>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.btn {
height: 50px;
display: flex;
align-items: center;
justify-content: center;
background-color: #409eff;
border-radius: 5px;
}
.btn-inner {
color: #fff;
}
.general-class {
margin-left: 40px;
padding: 10px;
width: 260px;
height: 160px;
background-color: antiquewhite;
}
.view-class {
margin: 20px 0 0 50px;
padding: 10px;
width: 240px;
height: 100px;
background-color: antiquewhite;
}
.view-class .text {
margin-top: 5px;
text-align: center;
}
.hover-class {
background-color: red;
}
</style>
名称 | 类型 | 兼容性 | 描述 |
---|---|---|---|
@click | (event: UniPointerEvent) => void | 手指触摸后马上离开。与tap相同,(推荐使用tap事件代替),冒泡事件 | |
@mousedown | (event: UniMouseEvent) => void | 鼠标在元素上点击后触发 | |
@mousemove | (event: UniMouseEvent) => void | 鼠标在元素上移动时触发 | |
@mouseup | (event: UniMouseEvent) => void | 鼠标主按钮在元素上松开时触发 | |
@touchstart | (event: UniTouchEvent) => void | 手指触摸动作开始,冒泡事件,event.type 值为 touchstart | |
@touchmove | (event: UniTouchEvent) => void | 手指触摸后移动,冒泡事件,event.type 值为 touchmove | |
@touchcancel | (event: UniTouchEvent) => void | 手指触摸动作被打断,如来电提醒,弹窗,冒泡事件,event.type 值为 touchcancel | |
@touchend | (event: UniTouchEvent) => void | 手指触摸动作结束,冒泡事件,event.type 值为 touchend | |
@tap | (event: UniPointerEvent) => void | 手指触摸后马上离开,冒泡事件 | |
@longpress | (event: UniTouchEvent) => void | 如果一个组件被绑定了 longpress 事件,那么当用户手指触摸后,超过350ms再离开会触发,冒泡事件 | |
@longtap | (event: UniTouchEvent) => void | 手指触摸后,超过350ms再离开(推荐使用 longpress 事件代替) | |
@transitionend | (event: UniEvent) => void | transition 效果结束时触发 | |
@fullscreenchange | (event: UniEvent) => void | 进入或退出全屏模式时触发 | |
@fullscreenerror | (event: UniEvent) => void | 进入或退出全屏模式失败时触发 |
Template
Script
<template>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view>
<page-head :title="title"></page-head>
<view class="uni-padding-wrap">
<view :id="generalId" :class="generalClass" :name="generalName" :title="generalTitle" :data-test="generalData"
:style="generalStyle" ref="general-target">
<text>id: {{ generalId }}</text>
<text>class: {{ generalClass }}</text>
<text>name: {{ generalName }}</text>
<text>title: {{ generalTitle }}</text>
<text>data-test: {{ generalData }}</text>
<text>style: {{ generalStyle }}</text>
</view>
<view class="btn btn-style uni-common-mt" @click="validateGeneralAttributes">
<text class="btn-inner">{{ validateGeneralAttrText }}</text>
</view>
<view class="btn btn-ref uni-common-mt" @click="changeHeight">
<text class="btn-inner">{{ changeHeightByRefText }}</text>
</view>
<view class="view-class" :hover-class="hoverClass" ref="view-target">
<text class="text">按下 50 ms 后背景变红</text>
<text class="text">抬起 400 ms 后背景恢复</text>
</view>
<view class="view-class" :hover-class="hoverClass" :hover-start-time="1000" :hover-stay-time="1000"
ref="view-target">
<text class="text">按下 1000 ms 后背景变红</text>
<text class="text">抬起 1000 ms 后背景恢复</text>
</view>
</view>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.btn {
height: 50px;
display: flex;
align-items: center;
justify-content: center;
background-color: #409eff;
border-radius: 5px;
}
.btn-inner {
color: #fff;
}
.general-class {
margin-left: 40px;
padding: 10px;
width: 260px;
height: 160px;
background-color: antiquewhite;
}
.view-class {
margin: 20px 0 0 50px;
padding: 10px;
width: 240px;
height: 100px;
background-color: antiquewhite;
}
.view-class .text {
margin-top: 5px;
text-align: center;
}
.hover-class {
background-color: red;
}
</style>
触摸事件包括:touchstart、touchmove、touchcancel、touchend 等。
在多点触摸的屏幕上,touch事件返回数组,包含了每个touch点对应的x、y坐标。
注意老版问题:uni-app x 4.0及以下版本手指按下后移动会取消tap/click事件的触发,即手指移动后抬起不会响应tap/click事件。
@transitionend
transition 效果结束时触发
安卓 3.93+ 版本开始支持
<template>
<image class="transition-transform" id="transition-transform" @transitionend="onEnd" src="/static/uni.png"></image>
</template>
<script>
export default {
data() {
return {}
},
onReady() {
var element = uni.getElementById('transition-transform')
element!.style.setProperty('transform', 'rotate(360deg)')
},
methods: {
onEnd() {
console.log("transition效果结束")
}
}
}
</script>
<style>
.transition-transform {
transition-duration: 2000ms;
transition-property: transform;
transform: rotate(0deg);
}
</style>
DOM事件主要有三个阶段:
捕获阶段
、目标阶段
和冒泡阶段
。
uvue
目前暂不支持事件的捕获阶段。
以点击事件为例,当触发点击时,
注意
虽然有3个阶段,但第2个阶段(“目标阶段”:事件到达了元素)并没有单独处理:捕获和冒泡阶段的处理程序都会在该阶段触发。
我们一般使用默认的事件注册机制,将事件注册到冒泡阶段,相对来说,大多数处理情况都在冒泡阶段。
在事件回调中,可以通过调用event.stopPropagation
方法阻止事件冒泡。
handleClick (event : UniPointerEvent) {
// 阻止继续冒泡.
event.stopPropagation();
}
在事件回调中,可以通过调用event.preventDefault
方法阻止默认行为。event.preventDefault
仅处理默认行为,事件冒泡不会被阻止。
<template>
<scroll-view style="flex: 1;">
<view style="width: 750rpx;height: 1750rpx;background-color: bisque;">
滑动框中区域修改进度并阻止滚动,滑动其余空白区域触发滚动
<view style="width: 750rpx;height: 40rpx; margin-top: 100rpx;border:5rpx;" @touchmove="slider">
<view ref="view1" style="background-color: chocolate;width: 0rpx;height: 30rpx;"></view>
</view>
</view>
</scroll-view>
</template>
<script>
export default {
data() {
return {
$view1Element: null as UniElement | null
}
},
onReady() {
this.$view1Element = this.$refs['view1'] as UniElement
},
methods: {
slider(e : TouchEvent) {
e.preventDefault() // 阻止外层scroll-view滚动行为
this.$view1Element!.style?.setProperty('width', e.touches[0].screenX);
}
}
}
</script>
click
或tap
事件,可能会出现事件丢失的情况。请升级新版调整
变更示例
<template>
<slider @change="sliderChange" />
</template>
<script>
export default {
data() {
return {
}
},
methods: {
// 变更之前类型为 SliderChangeEvent
// sliderChange(e : SliderChangeEvent) {
// }
// 变更之后类型为 UniSliderChangeEvent
sliderChange(e : UniSliderChangeEvent) {
}
}
}
</script>
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | 事件的名称 |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | 事件的名称 |
eventInit | any | 是 | - | - | 事件初始参数。支持字段:bubbles 表明该事件是否冒泡。可选,默认为false;cancelable 表明该事件是否可以被取消。可选,默认为false。 |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
bubbles | boolean | 是 | - | - | 是否冒泡 |
cancelable | boolean | 是 | - | - | 是否可以取消 |
type | string | 是 | - | - | 事件类型 |
target | UniElement | 否 | - | - | 触发事件的组件 |
currentTarget | UniElement | 否 | - | - | 当前组件 |
timeStamp | number | 是 | - | - | 事件发生时的时间戳 |
阻止当前事件的进一步传播
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | - | - | - |
阻止当前事件的默认行为
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
4.0 | - | 3.9 | x | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | - |
detail | T | 是 | - | - | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | - |
options | any | 是 | - | - | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
detail | T | 是 | - | - | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
clientX | number | 是 | - | - | 相对于页面可显示区域左边的距离 |
clientY | number | 是 | - | - | 相对于页面可显示区域顶部的距离 |
x | number | 是 | - | - | 相对于页面可显示区域左边的距离,同clientX |
y | number | 是 | - | - | 相对于页面可显示区域顶部的距离,同clientY |
pageX | number | 是 | - | - | 相对于屏幕左边的距离,包括滚动距离。 |
pageY | number | 是 | - | - | 相对于屏幕顶部的距离,包括滚动距离。 |
screenX | number | 是 | - | - | 相对于屏幕左边的距离,不包括滚动距离。 |
screenY | number | 是 | - | - | 相对于屏幕顶部的距离,不包括滚动距离。 |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 | ||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
touches | Array<UniTouch> | 是 | - | - | 当前停留在屏幕中的触摸点信息的数组 | ||||||||||||||||||||||||||||||||||||||||||||||||||||||
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
changedTouches | Array<UniTouch> | 是 | - | - | 当前变化的触摸点信息的数组 | ||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
UniTouchEvent 的 type 类型包括:touchstart、touchmove、touchend、touchcancel、longpress。
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
clientX | number | 是 | - | - | 相对于页面可显示区域左边的距离 |
clientY | number | 是 | - | - | 相对于页面可显示区域顶部的距离 |
identifier | number | 是 | - | - | 触摸点的标识符。这个值在这根手指所引发的所有事件中保持一致,直到手指抬起。 |
pageX | number | 是 | - | - | 相对于屏幕左边的距离,包括滚动距离。 |
pageY | number | 是 | - | - | 相对于屏幕顶部的距离,包括滚动距离。 |
screenX | number | 是 | - | - | 相对于屏幕左边的距离,不包括滚动距离。 |
screenY | number | 是 | - | - | 相对于屏幕顶部的距离,不包括滚动距离。 |
force | number | 否 | - | - | 返回当前触摸点按下的压力大小 |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
clientX | number | 是 | - | - | 相对于页面可显示区域左边的距离 |
clientY | number | 是 | - | - | 相对于页面可显示区域顶部的距离 |
x | number | 是 | - | - | 相对于页面可显示区域左边的距离,同clientX |
y | number | 是 | - | - | 相对于页面可显示区域顶部的距离,同clientY |
pageX | number | 是 | - | - | 相对于屏幕左边的距离,包括滚动距离。 |
pageY | number | 是 | - | - | 相对于屏幕顶部的距离,包括滚动距离。 |
screenX | number | 是 | - | - | 相对于屏幕左边的距离,不包括滚动距离。 |
screenY | number | 是 | - | - | 相对于屏幕顶部的距离,不包括滚动距离。 |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
keyCode | number | 是 | - | - | - |
keyType | string | 是 | - | - | - |
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | - | - | - |
native-view自定义事件
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | - |
detail | any | 是 | - | - | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | - |
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
type | string | 是 | - | - | 事件类型 |
detail | UTSJSONObject | 是 | - | - | - |
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | 4.31 | 4.31 | 4.61 |
通用事件
临时方案,规避组件Event接口无法直接继承UniEvent的问题
名称 | 类型 | 必备 | 默认值 | 兼容性 | 描述 |
---|---|---|---|---|---|
bubbles | boolean | 是 | - | - | 是否冒泡 |
cancelable | boolean | 是 | - | - | 是否可以取消 |
type | string | 是 | - | - | 事件类型 |
target | UniElement | 否 | - | - | 触发事件的组件 |
currentTarget | UniElement | 否 | - | - | 当前组件 |
timeStamp | Long | 是 | - | - | 事件发生时的时间戳 |
timeStamp | number | 是 | - | - | 事件发生时的时间戳 |
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | - | - | - |
阻止当前事件的进一步传播
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | - | - | - |
阻止当前事件的默认行为
Web | 微信小程序 | Android | iOS | HarmonyOS |
---|---|---|---|---|
- | - | - | - | - |